本文将介绍如何在 SpringBoot 项目中使用 SpringBoot JPA 。
一、引入依赖
在 pom.xml 中添加依赖如下:
1 2 3 4 5 6 7 8 9
| <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency>
<dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> </dependency>
|
二、添加配置文件
在 application.properties 中,添加配置信息如下:
1 2 3 4 5 6 7
| spring.datasource.url=jdbc:mysql://localhost:3306/数据库名 spring.datasource.username=root spring.datasource.password=root spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver spring.jpa.properties.hibernate.hbm2ddl.auto=update spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.MySQL5InnoDBDialect spring.jpa.show-sql=true
|
三、新建实体类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| @Entity public class User implements Serializable {
private static final long serialVersionUID = 1L;
@Id @GeneratedValue private Long id;
@Column(nullable = false) private String userName;
@Column(nullable = false) private String passWord;
@Column(nullable = false) private String email;
}
|
四、新建 repository
定义接口,继承 JpaRepository 即可。
JpaRepository<T, ID>
中,
1 2 3 4
| @Repository public interface UserRepository extends JpaRepository<User, Long> { }
|
五、父类方法
继承 JpaRepository 之后,repository 便拥有父类的一系列方法,例如:
1. 测试 - 保存数据
1 2 3 4 5 6 7 8 9 10 11 12
| @SpringBootTest public class UserRepositoryTest {
@Autowired private UserRepository userRepository;
@Test public void testSave() { userRepository.save(new User("小明", "111111", "1@qq.com")); } }
|
保存前:
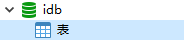
保存后:
JPA 会自己建立 table,并将数据插入。

2. 测试 - 查找数据
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| @SpringBootTest public class UserRepositoryTest {
@Autowired private UserRepository userRepository;
@Test public void testSave() { for (User user : userRepository.findAll()) { System.out.println(user); } } }
|
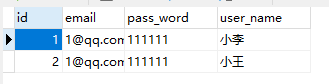

3. 分页查询
SpringBoot JPA 自身便支持分页功能,其具体做法是:
六、自定义方法
1. 简单方法
SpringBoot JPA 支持根据方法名来指定匹配 SQL、执行方法,其语法为:
findBy属性名()
findBy属性名1Or属性名2()
findBy属性名1IgnoreCase
findBy属性名1OrderBy属性名2()
deleteBy属性名()
count属性名()
只需要在 repository 中定义方法,便可以通过 repository 直接调用,而不用关心如何实现。
2. 限制查询
在 repository 中定义方法,例如:
查询根据属性名匹配的前 n 个元素:findFirstnBy属性名()
3. 自定义 SQL 查询
在 repository 中定义方法,
- 在希望自定义 SQL 的方法上用
@Query(SQL语句)
注解
- 在涉及到删除和修改的方法上
@Modifying
注解
4. 连表查询
待更新
参考