本文将介绍 Jedis,Java 中用于连接 Redis 的依赖。
一、Redis
具体请看:
分类 - Redis
二、示例
此处以 Maven 项目为例
1. 导入依赖
在 pom.xml 中,添加信息如下:
1 2 3 4 5 6 7
| <dependencies> <dependency> <groupId>redis.clients</groupId> <artifactId>jedis</artifactId> <version>版本号</version> </dependency> </dependencies>
|
2. 简单使用
Jedis 将 Redis 中的命令都实现为方法,像输入命令一样调用方法即可。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| public class testJedis { public static void main(String[] args) { Jedis jedis = new Jedis("127.0.0.1", 6379); System.out.println(jedis.ping()); jedis.set("name", "Tom"); System.out.println(jedis.get("name")); jedis.close(); } }
|
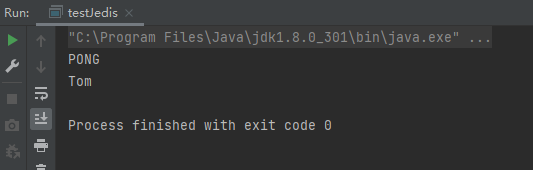
三、连接池
Jedis 集成了连接池,带连接池的 Redis 工具类封装示例如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80
| public class RedisUtil {
private static String ADDR = ""; private static int PORT = 6379; private static String AUTH = "123456"; private static int MAX_ACTIVE = 1024; private static int MAX_IDLE = 200; private static int MAX_WAIT = 10000; private static int TIMEOUT = 10000; private static boolean TEST_ON_BORROW = true; public static final int DEFAULT_DATABASE = 0;
private static JedisPool jedisPool = null;
static {
try {
JedisPoolConfig config = new JedisPoolConfig(); config.setMaxTotal(MAX_ACTIVE); config.setMaxIdle(MAX_IDLE); config.setMaxWaitMillis(MAX_WAIT); config.setTestOnBorrow(TEST_ON_BORROW); jedisPool = new JedisPool(config, ADDR, PORT, TIMEOUT,AUTH,DEFAULT_DATABASE);
} catch (Exception e) {
e.printStackTrace(); }
}
public synchronized static Jedis getJedis() {
try {
if (jedisPool != null) { Jedis resource = jedisPool.getResource(); System.out.println("redis--服务正在运行: "+resource.ping()); return resource; } else { return null; }
} catch (Exception e) { e.printStackTrace(); return null; }
}
public static void returnResource(final Jedis jedis) { if(jedis != null) { jedisPool.returnResource(jedis); } } }
|
参考